Dropout은 overfitting을 막기 위한 regularization 방법이다.
Dropout에는 크게 두 가지 방법이 있다고 볼 수 있다.
Dropout
여기서 설명하는 Dropout은 Dropout: A Simple Way to Prevent Neural Networks from Overfitting 논문에서 나온 형태이다.
- training time에는 drop_rate의 확률로 노드의 출력을 0으로 만든다.
- PyTorch, Keras에서는 drop 확률(PyTorch: p / Keras: rate)을 사용한다.
- 원 논문에서 사용하는 확률 p는 drop할 확률이 아니라 다음 노드로 출력을 전달할 확률이다.
- test time에는 출력을 (1 - drop_rate) 만큼 곱해주어 training time 때의 기대 출력과 동일하게 만들어준다.
- e.g.) drop_rate가 0.4이면 출력에 0.6을 곱해준다.
- 원 논문에서 얘기하는 p로 설명했을 때는 출력에 p를 곱해줘야한다.
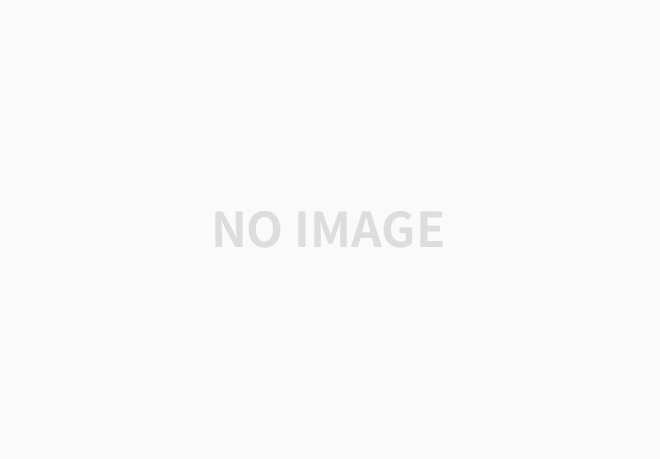
# dropout 예제 코드 import numpy as np def dropout(inputs, drop_rate, training): assert 0 <= drop_rate < 1 if training: mask = drop_rate < np.random.uniform(0, 1, inputs.shape) return inputs * mask return inputs * (1-drop_rate)
Inverted Dropout
앞서 살펴본 Dropout의 변형된 형태로 PyTorch, Keras 모두 사용하고 있는 Dropout 방법이다.
(Dropout doc 참고: PyTorch, Keras)
- training time에는 drop_rate의 확률로 노드의 출력을 0으로 만들고(비활성화),
출력이 0이 아닌(활성화된) 노드의 출력에 1 / (1 - drop_rate)를 곱해주어 그 값을 키운다.- 앞서 Dropout 에서 test time때 (1 - drop_rate)를 곱해준 것 처럼,
inverted dropout의 test time 때 기대 출력과 동일하게 만들어 주기 위함인 것 같다.
- 앞서 Dropout 에서 test time때 (1 - drop_rate)를 곱해준 것 처럼,
- test time에는 아무런 연산 없이 그대로 출력 해준다.
- test time에 연산을 없앰으로써 모델의 테스트 성능을 높여준다.
# 예제 코드 import numpy as np def inverted_dropout(inputs, drop_rate, training): assert 0 <= drop_rate < 1 if training: mask = drop_rate < np.random.uniform(0, 1, inputs.shape) masked_input = inputs * mask return masked_input / (1.0 - drop_rate) return inputs
참고
'Machine Learning > Deep Learning' 카테고리의 다른 글
CNN Filter Visualization (0) | 2024.06.16 |
---|---|
CNN에서 layer가 깊어질 수록 channel size를 키우는 이유 (0) | 2024.05.31 |
Manifold와 뉴럴 네트워크 학습 (0) | 2024.05.20 |
Non zero-centered activation function과 학습 비효율 (1) | 2024.05.01 |
ReLU의 활성 함수으로써의 사용과 미분 가능성 (0) | 2024.04.23 |
댓글